PHP Performance Optimization on Strings,Arrays,Errors,OOP
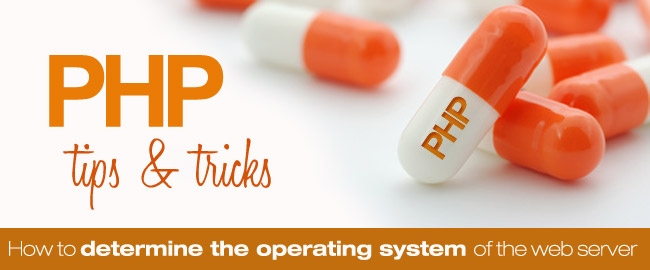
Here are some performance/optimization tips, tricks and best practices to keep in mind when developing in PHP.
The list will be updated on a regular basis, so check back for updates
Strings
• Use str_replace() instead of preg_replace() (regular expressions); Regular expressions are slower (but powerful), so only use them if necessary. In saying that, if you are calling trim(str_replace(explode($somestring))) or something of that magnitude, consider using regular expressions as it is probably faster! Ultimately, do what is quickest for you to develop and easiest to maintain in most cases, as the trade-off in efficiency is insignificantly minimal.
• Use the sprintf() function instead of variables contained in double quotes; It's about 10x faster (Cite).
• PCRE regex is quicker than EREG; however as mentioned previously use other functions such as str_replace(), $strpos(), etc...as those are faster than regular expressions.
Error Management
• Error suppression "@" is expensive; Where applicable, check with functions before the operation such as isset() or file_exists() depending on the situation.
• Error Messages in general are expensive; as it is IO related. Code with best practices in mind and avoid PHP Errors and Notices. Turn on error_reporting(E_ALL) for all errors and fix up all the errors and notices displayed.
Database
• Don't forget to close your database connection when you are done with them.
Arrays
• Always specify the Array Elements with single quotes; $array['hello'] v.s. $array[hello]; Although both will work, the latter will require PHP to look for the constant "hello" before using it as the string "hello".
• Arrays are much faster than objects; and array operations are faster than object operations. Prefer to use arrays and use objects only when it impacts code maintainability and/or legibility.
• isset($array['element']) is much faster than iterating through the array (using methods such as in_array()).
Object Oriented Programming
• Static methods are faster than non-static methods; Use static where applicable, given the object has not been initialized (around 33% faster). If an object has already been initialized, then calling the non-static method on the initialized object is actually faster than the static one (static around 14% slower).
• Avoid writing useless setter and getter methods. If there is no genuine reasons of encapsulating the object variables, then don't. It may be up to 2x faster to access the object variable directly.
• Static methods are faster than non-static (member) functions. Use static where possible can result in better performance, however should not compromise and restrict your end goal.
• Incrementing and decrementing a local variable is much faster than a global variable; it is also slow to increment/decrement an object property.
• Incrementing an undefined variable can be up to 10x slower than a pre-initialized one; always define variables you are going to use.
• Methods defined locally in the object executes faster than inherited functions in base class(es).
• OOP is good practice but if the code can be done in a procedural manner, it will be faster and won't have the overheads of object calls.
Comments 0