PHP Fibonacci Series printing
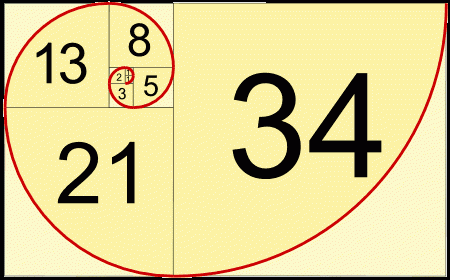
A Fibonacci Series of numbers will look like
0 , 1 , 1 , 2 , 3 , 5 , 8 , 13 , 21 , 34 , 55
First two Fibonacci numbers are 0 and 1 . After that each number will be the sum of previous two numbers . Here we can see that third Fibonacci number is 1 ( sum of first two numbers 0 + 1 ) , and fourth Fibonacci number will be the sum of third and second number ( 1 + 1 = 2 ) , and fifth Fibonacci number will be the sum of fourth and third number ( 2 + 1 = 3 ). The series will go like that infinity .
Fibonacci number using recursion
function fibonacci($n){
$iResult = 1;
if ($n == 0){
$iResult = 0;
}else if ($n >= 2){
$iResult = fibonacci($n - 1) + fibonacci($n - 2);
}else if ($n < 0){
$iResult = -1;
}
return $iResult;
}
//---print fibonacci sequence length 10
for($i=0;$i<=10;$i++){
echo fibonacci($i).",";
}
Fibonacci number without using recursion
function fibonacci($n) {
if($n <0)return -1;
if ($n == 0)return 0;
if($n == 1 || $n == 2)return 1;
$int1 = 1;
$int2 = 1;
$fib = 0;
//start from n==3
for($i=1; $i<=$n-2; $i++ ){
$fib = $int1 + $int2;
//swap the values out:
$int2 = $int1;
$int1 = $fib;
}
return $fib;
}
//---print fibonacci sequence length 10
for($i=0;$i<=10;$i++){
echo fibonacci($i).",";
}
Comments 0